|
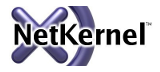
NetKernel News Volume 3 Issue 34
August 24th 2012
What's new this week?
- Repository Updates
- Resource Oriented Analysis and Design - Part 1
- Tic Tac Toe Resource Model
- Preview: Documentation Visualization
- NetKernel West 2013
Catch up on last week's news here
Repository Updates
No updates this week. Steady as you are...
Resource Oriented Analysis and Design - Part 1
First in a series of articles taking a well understood domain problem and casting it as an ROC solution.
Background
People who have learned ROC will tell you "ROC is not difficult, just different". But over the last year I've seen a number of cases where developers immersed in state-of-the-art best practices for "coding" have spectacularly bounced off. Whilst equally, in the same time I've seen people with no experience of ROC rapidly develop and deploy to production massively scaled heavily loaded mission critical systems that "just work".
The latter cases were achieved by going with the flow, relaxing and letting the system take the strain and, fundamentally embracing "Composition" (the second of the 3C's). The bounces seem to happen when the initial starting assumption is "Construct" - ie "we build software by writing code".
The challenge for anyone embracing ROC is that this latter proposition is actually the current industry-best-practice. For example, we see it in the practices of Test Driven Development (TDD)... before you do anything write (code) a test which fails then write (code) some code that makes the test-code pass. repeat... code test-code, code code, code test-code, code code...
One of our challenges in the next phase of tooling in NetKernel is to introduce a set of technical measures that will attempt to meet half-way the working practices of "regular coders". In short to make things feel more like they're used to them feeling. Whether its IDE integration, testing frameworks, classpaths etc. (See below for an example of concrete steps that are underway).
But there is a fundamental dilemma - since to make ROC rigidly fit into the orthodox tooling would be to constrain its power and flexibility. It is precisely because "Constraint" (the third C) is last in the three C's that ROC solutions are malleable, adaptive, fast to create, easy to evolve. With ROC anyone who's ever learned it will tell you "change is cheap". (the fourth and fifth C's!).
Anyway - that is a little context for what follows, and it has resulted in me reading around and examining the "classical paradigm".
Recently, I came across an often cited example called "TDD as if you meant it"...
http://gojko.net/2009/08/02/tdd-as-if-you-meant-it-revisited/
An article about a workshop teaching the TDD methodology. I realised that the domain problem which is chosen, to implement "Tic Tac Toe" ("Noughts and Crosses", to Europeans), would equally serve as an exercise in showing how to think and work in ROC. Also, since the teams in the article have provided the source code of their solutions, maybe we can get a sense of comparison.
What follows is, as near as I can make it, a stream of consciousness description of my approach to the TicTacToe problem. Along the way I hope to show the balance between C1 and C2 (construct and compose) and how C3 (constraint) is used sparingly and late in the process. In doing so I also hope to show, what I've recently come to think of as, ROC's "Relevant Testing".
Who knows, maybe I'll pull it off, maybe I'll crash and burn? As I write I'm winging it and have written absolutely no code. All I've done is spend half an hour thinking about the problem and the resources. So lets get started...
Tic Tac Toe Resource Model
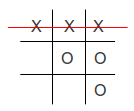
The game of Tic Tac Toe (Noughts and Crosses) is one you no doubt learned when you were a young kid. A two-dimensional array provides a grid of 9-cells in which you may mark your token (a nought O or a cross X). Each player takes a turn to mark a cell. The winner is the first to get three consecutive tokens in a straight line.
A mathematician would be able to tell you that there are a couple of hundred thousand possible games. The first player to go has a two-to-one statistical advantage! And, rather sad for your inner child, achieving a draw is assured unless your opponent makes a mistake.
But you know the rules, that's the whole point. We don't need to spend any time defining the domain problem.
Classical Approach
When you read the TDD article you will see that they also waste no time on the model - but instead get to the definition of the constraints. Which we'll quote directly (I changed their term "field" to cell since I just think its the more precise technical name of a region of a lattice grid)...
- a game is over when all cells are taken
- a game is over when all cells in a column are taken by a player
- a game is over when all cells in a row are taken by a player
- a game is over when all cells in a diagonal are taken by a player
- a player can take a cell if not already taken
- players take turns taking cells until the game is over
This is a Constraints-first approach to defining the problem. It is unstated and implicit that there is state associated with a cell and indeed the whole playing grid. There are also a whole set of implicit and presumptive terms (row, column, diagonal) - of course we know what these mean since we know the problem intimately (but don't forget the implicit presumptions going on at this point).
No doubt those trained in Object Oriented design are already envisaging a Cell class with associated getters and setters, a Game class containing a structured set of Cell members and an interface of methods which could be called to determine if the constraints above are applied etc etc. Lets call this the "classical approach" - we'll undoubtedly come back to this later in the series of articles.
So now lets switch to the ROC perspective. At first it might look quite similar, but after only a very short transition we start to look at things in quite a different way...
ROC Approach
First we start by thinking about the resources and their possible state. What we're aiming to find are the Sets and the memberships of sets and any associated compositional Sets (composites).
Here is a diagram and definitions of the resources of the problem (this is a tidied up copy of something I sketched up on the whiteboard in ten minutes)...
Resource
The first thing we notice is that a Cell resource is the "atom of state". We see that it may have states of "NULL, O and X". So far so very like OO-modelling.
But the very next thing to notice is that every Cell has an identity. In fact I started by thinking - "what is the name of a cell resource". I have not worried about an ROC URI name (we can choose our naming convention later) but what I am saying is that a cell has an identity Cell(x,y) and x and y are grid reference co-ordinates.
So lets define Cell(0,0) as the identity of the resource "top left cell" and Cell(2,2) is the identity of the resource "bottom right cell".
Composite Resources
We know from our knowledge of the domain problem that the outcome of the game depends upon the concepts of "row", "column" and "diagonal" - indeed to win requires that the state of one these should be all X's or all O's. Immediately we have identified three new sets of resources and so we can give them identities. Row(y), Column(x) and Diagonal(i).
But with a little more thought we realise that Rows, Columns and Diagonals are not atomic resources. They are composite resources consisting of small sets of atomic Cell(x,y) resources. In short we see that they are subsets of the set of all Cells{ }.
Immediately we realise that we now need to define a new composite resource Cells{ ... } comprising a set of Cell(x,y) resources and which therefore allows us to give an identity to any subset of cells (hang with it - it will become very clear in a second).
So when we refer to Row(0) what we're really doing is talking about the identity of a set of cells. Similarly when we talk about Column(1) this is another set of cells. Row, Column and Diagonal are just aliases to specific subsets of Cells{...}.
It follows that we don't need to "write any code" for Row, Column or Diagonal - we can just declare a mapping from the aliased identity to the explicit identity. So...
Column(x) is an alias for Cells{ Cell(x,0), Cell(x,1), Cell(x,2) }
which, to be explicit means that ...
Column(1) is an alias for Cells{ Cell(1,0), Cell(1,1), Cell(1,2) }
similarly, you can see in the diagram we can define alias mappings for Row(y) and the Diagonals.
Pause a moment and think about this.
- We have defined one set of atomic resources: Cell(x,y)
- We have defined one set of composite resources: Cells{...} (which references atomic Cell(x,y) resources).
- We have defined three sets of composite resources that have "meaning to our game" but which are really just aliases for subsets of the Cells{...} resource set.
Maybe we might want to start to think about new games in which the concept of "The Corners" has meaning - no problem its just another named subset of Cells{}... but lets not get ahead of ourselves, we've not written TicTacToe yet...
What we're doing here is saying "giving things identity is powerful" it allows us to refer to things collectively - that is, it allows us to deal with whole sets rather than small parts. (Remember when in a previous newsletter I said "the bag is man's greatest invention", for this same reason? We just rediscovered this).
OK now we've conceived the fundamental resource model we can build on it to define some more meta resources that have relevance to the game of TicTacToe...
We know that its important to know if the game has been won. By sticking to the mindset of "Sets of Cells" we realise that there is a meta resource which we can call the WinningSet - it is a composite resource and is another subset of Cells{...}.
At the start of the game the WinningSet is empty. When the game proceeds, eventually we will want to hold the state of the winning row, column or diagonal - the WinningSet will be made real. But we don't need to bother ourselves with writing any code to do this yet - its sufficient for the moment that we have the concept of "WinningSet" and understand it as an unreified subset of Cells{...}.
Equally we can see that having a history of played moves is a useful resource. Again it is a meta resource and is just a list of Cells (another subset of Cells{...}).
So now we can define the GameOver resource - this is true if and only if the WinningSet is not empty or there is no FreeSpace left to play. We already understand what the WinningSet is and its easy to see that the FreeSpace resource is just 9 minus the size of the History set.
Finally we have a resource which is really alien to the classical domain. When we play a move we need to decide if it was the winning move. So we need to check to see if it fills a row or column or diagonal. We can define the CheckSet(x,y) for a Cell(x,y) as being the identities of the sets we need to go and check. So the resource CheckSet is a "linked data" resource!
Say we play Cell(0,0) then CheckSet(0,0) would be the resource:
{ Column(0), Row(0), Diagonal(0) }
The set of identities of the set of resources we need to know the state of to see if we've reified the WinningSet.
This last concept of a set of linked data resources is not so far from the Cells{...} (also linked data resource!) but you might be beginning to feel a bit light headed!
Next time we'll start to implement the solution and it will crystallize. But you may already be beginning to sense that without writing any code we're on the path to a normalized solution - the logic we will need to execute to play the game will be the bare minimum. (You might want to peak ahead and compare and contrast where we're leading with the "repeated iterations of cells" designs seen in the OO of the classical solutions in the TDD exercise).
Further Reading
You might be starting to see that the resources that are relevant to our game are really just metadata. It will probably pay back to read this quite detailed discussion of how ROC allows us to create a very hardcore definition of what metadata actually means...
If you are an experienced NK developer - you can probably knock up a solution to the problem from these resource definitions. If you want to submit a solution before I talk about doing this next week - we could share the solutions for reference and discussion. Just as with the OO/TDD teams - there is no one answer to the problem.
Next time - we might or might not need to write some code... ;-)
Preview: Documentation Visualization
Tony has a project on the go to make discovery and navigation of the documentation more powerful. He's given a sneak peak of a new tool we'll be releasing soon...
http://durablescope.blogspot.co.uk/2012/08/mindmap-of-netkernel-universe.html
NetKernel West 2013
Several people have recently asked if we're planning a conference. To which the answer is: "planning" would be too strong a word - but heck, yeah, why not...
So here's the "plan"...
Date: lets say spring 2013 to give everyone some lead time.
Location: USA. Those who've been to any of the previous three conferences will know we have exacting requirements. It has to be somewhere young, cool and hip (college towns have been good to us so far), within reasonable travel from a decent airport, *not* be a corporate hotel and (purely coincidentally) have a thriving local brew-scene. Any ideas?
Format: Shall we stick with the one or two day pre-conference bootcamp followed by two-day main conference?
Content: Now you could come along and listen to me go on and on about the esoteric nature of reality and the relation between resource spaces and chrono-synclastic infundibulae*... But its time to get real. To share experiences. To establish common practice. To get a shared perspective of how ROC fits in the IT landscape....
What we as a community all need are your presentations describing your experience, your practices, your patterns, your stories...
So get thinking and send me a short proposal. All selected speakers will be rewarded with some shameless give-away (can we do your own weight in beer?)...
Sounds like a plan... see you in 2013...
*Courtesy of Kurt Vonnegut and pointed out by B. Sletten Esq.
Have a great weekend.
Comments
Please feel free to comment on the NetKernel Forum
Follow on Twitter:
@pjr1060 for day-to-day NK/ROC updates
@netkernel for announcements
@tab1060 for the hard-core stuff
To subscribe for news and alerts
Join the NetKernel Portal to get news, announcements and extra features.
NetKernel will ROC your world
![]() |